I love HTM for it’s simplicity. HTM allows you to write JSX like syntax but without Webpack, and other complexities. The great thing about HTM is that it’s just 700 bytes. That’s right. When you setup a Webpack environment it’s very easy to reach 200MB+.
Webpack might be good for complex applications but I wouldn’t want so much of configurations for a simple WordPress add-on that I can complete in an evening.
Installation
Get the HTM code from here:
https://unpkg.com/htm@2.0.0/preact/standalone.mjs
It is very important to let HTM know which function to use to create the elements. While developing Gutenberg block we use `wp.element.createElement` function.
const html = htm.bind( wp.element.createElement );
Then you can easily write JSX like code:
return html`
<div >
<${Fragment}>
<${BlockControls}>
<${SelectControl} class="gch_lang_selector" value=${language} onChange=${editLanguage} label='' options=${available_languages} />
<${SelectControl} class="gch_style_selector" value=${style} onChange=${editStyle} label='' options=${HIGHLIGHTJS_STYLES} />
<//>
<textarea class='${props.className} gch_ta' onChange=${editTA}>${code}</textarea>
<//>
</div>`;
The HTM syntax
Unlike JSX, HTM makes use of Javascript template literals. a In JSX you don’t need to wrap your code in a string. You can write it like this:
return(<div>
<h1>The heading</h1>
</div>
)
It’s a bit different with HTM
return(html`<div>
<h1>The heading</h1>
</div>`
)
How HTM is different from JSX
JSX | HTM |
<div></div> | html`<div></div>` |
<Footer>Footer Content</Footer> | html`<${Footer}>Footer Content<//>` |
<div className=’myCLass’> | html`<div class=’myCLass’> |
Catch to avoid
There are a few things you should absolutely know:
1, Although HTM code looks similar to JSX and HTML, it’s neither of them. Mind those differences.
2, When you start a custom tag, end it with Component end-tag. Not doing so caused syntax error in my case. Which took me some time to figure out. Example:
<${Footer}>footer content<//>
Syntax Highlighting
When I started writing code using HTM one thing that made me uncomfortable was that there was no Syntax highlighting inside the template literals. The code looked banal
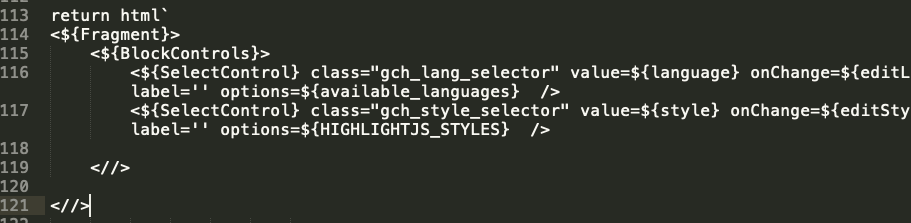
I couldn’t write code like this and you can’t also. The good news is that you have
- Lit-html extension for VS Code
- vim-jsx-pretty plugin for Vim
- For sublime you can try this trick.
Next..
I’d recommend you to read the HTM readme document here. You can also check out the source code my WordPress plugin for inspiration.